In almost every Arduino tutorial we’ve written we’ve used serial output for either printing text to terminal or plotting values. It is also invaluable as a debugging tool. We have rarely written about serial input, however, which is what this post is about.
What Can Serial Input Be Used for?
Check For Data Input with Serial.available This next sketch uses Serial.available to check for input from your serial monitor. Fundamentally, we will stay in setup until we receive an input from the Arduino serial monitor. Go ahead and upload this sketch.
- How to input NUMBERS through Arduino serial.monitor??? Convert that to intiger //input number through serial monitor and blink led for the.
- Hi forum, we are trying to make a school project with the arduino mega 2560 we want to control a servo from serial.monitor.we want to give a angle(a namber) to servo from keyboard and the servo to go here.
The possibilites with serial inputs are endless. Maybe you want to display text on an LCD display, punch in numbers to controll LEDs, control motor movement with arrow keys or send commands to decide which functions to call. No matter what you decide to use it for, your system reaches a higher level of interactivity.
How?
Using serial inputs is not much more complex than serial output. To send characters over serial from your computer to the Arduino just open the serial monitor and type something in the field next to the Send button. Press the Send button or the Enter key on your keyboard to send.
Coding wise, let’s dive into an example.
There are two important functions related to the serial input in the code above, and that is Serial.available()
and Serial.read()
.
Serial.available()
returns the number of characters (i.e. bytes of data) which have arrived in the serial buffer and that are ready to be read.
Serial.read()
returns the first (oldest) character in the buffer and removes that byte of data from the buffer. So when all the bytes of data are read and no new serial data have arrived, the buffer is empty and Serial.available()
will return 0.
If we send more than one character over serial with this code, the output will look like this:
But what if you want to send more than one character in handle it in a sensible way? No problemo!
Here we’ve introduced the readStringUntil()
function, which makes it possible to combine all the characters in the sent message into a single Arduino string. In this case we’re waiting for the n
character, which is the newline character that comes at the end of a string sent in the Arduino serial monitor.
Sending Commands
A more usable scenario could be to send commands to the Arduino. Depending on what you send, the Arduino will perform different task. Here’s a somewhat abstract example on how to do this:
Notice the use of the equals()
function. This is a function in the Arduino String class which returns true
if the string in question is equal to the parameter string.
Summary
Serial inputs can be very useful in your Arduino project. It’s a bit more complex than serial output, but not by much! The key functions are Serial.available()
and Serial.read()
.
We briefly touched upon this topic in our post about Arduino serial input, but inspired by this excellent tutorial by Stephen Brennan we thought it would be fun to create a more rigid command line interface (aka. CLI) for the Arduino than what we did in the serial input post. We recommend you read Brennan’s tutorial regardless since it contains a lot of important info about this topic and programming in general which we won’t mention here.
Having a CLI on an embedded system like the one in this blog post is quite different from a shell in Linux for instance since you usually don’t have an OS to back you up with multiprocessing and all that jazz. There are ways around this, but we’ve kept everything sequential for simplicity’s sake in this example. This means that several tasks can’t run simultaneously and that one task has to finish before starting a new one.
What our CLI looks like in Arduino’s serial monitor.
The purpose of a CLI like this on an Arduino is to have the ability to send text commands to it during runtime to execute certain tasks, such as controlling a servo, displaying text on a screen or launching a rocket.
We’re basically going to go quickly go through the code in chunks in this post. But first we’ll briefly explain what our program does.
Our CLI
The primary purpose of this specific code is to control the on-board LED tied to pin 13 on the Arduino. You can either turn it on, off or having it blink 10 times at a given frequency. We’ve also included a help command which explains what each command does as well as an exit command which just puts the program in a while(1)
state.
The Code
The code itself is just over 200 lines where many are just pure printing functions, so the core functionality is not that complex.
Setting Things up

Arduino Serial Monitor Output
Let’s start with the global variables, defines and the setup()
and loop()
functions.
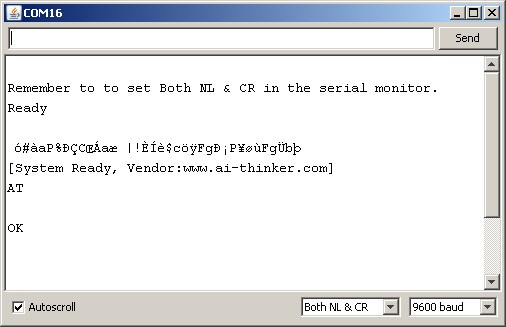
When the user enters a command, the program will compare it to the strings in commands_str
and call the function in commands_func
with the same index.
Top-level Functions
The cli_init()
function which is called in setup()
just displays a short welcome message while my_cli()
is where the the magic starts to happen.
The Meat of the CLI
Three important functions are called within my_cli()
. These are:
read_line()
– this waits for input from the user and stores it in theline
string.parse_line()
– this divides the input into arguments and stores them in theargs
list. The delimiter used is a space.execute()
– this calls the correct function based on the user’s input.
The memset functions reset the line
string and the args
list to zero.
In the read_line()
function we don’t really guard against very long inputs. It is still stored in the line_string
Arduino string. This means that very long inputs can still mess with our memory.
In the parse_line()
function, strtok()
is the real work horse. This function splits a string into several substrings where it finds the delimiter specified, which in our case is a white space. Read more about strtok()
here.
The execute()
function is pretty straight forward. Notice that the function in commands_func
at index i
is called when returning from this function within the for loop.
Getting Help
Here you have all the help functionality. When the user types help and press enter, the cmd_help()
function is called. This function checks what the user wrote after help and call the correct function based on that. help_help()
, help_led()
and help_exit()
are just functions that print stuff to the terminal.
LED Control and a Silly Exit Function
The cmd_led()
function is the only one that uses three arguments. The third argument (blinking frequency) is used directly in the calculation and not just to to define what function to call.
The cmd_exit()
doesn’t check other arguments, so no matter what you write after exit, it will run normally.
Summary
The most important thing to learn from this blog post is the core building blocks my_cli()
, read_line()
, parse_line()
and execute()
and the general flow of the program. To make it possible for commands to run “simultaneously” you will have to make some fundamental changes to the program flow and make the read function non-blocking.
Arduino Input Via Serial Monitor
Also, as already mentioned, make sure you take a look at Brennan’s tutorial.